Seamlessly Integrating TypeScript with React and Vite
Master the art of integrating TypeScript with React and Vite for cleaner, more robust code!
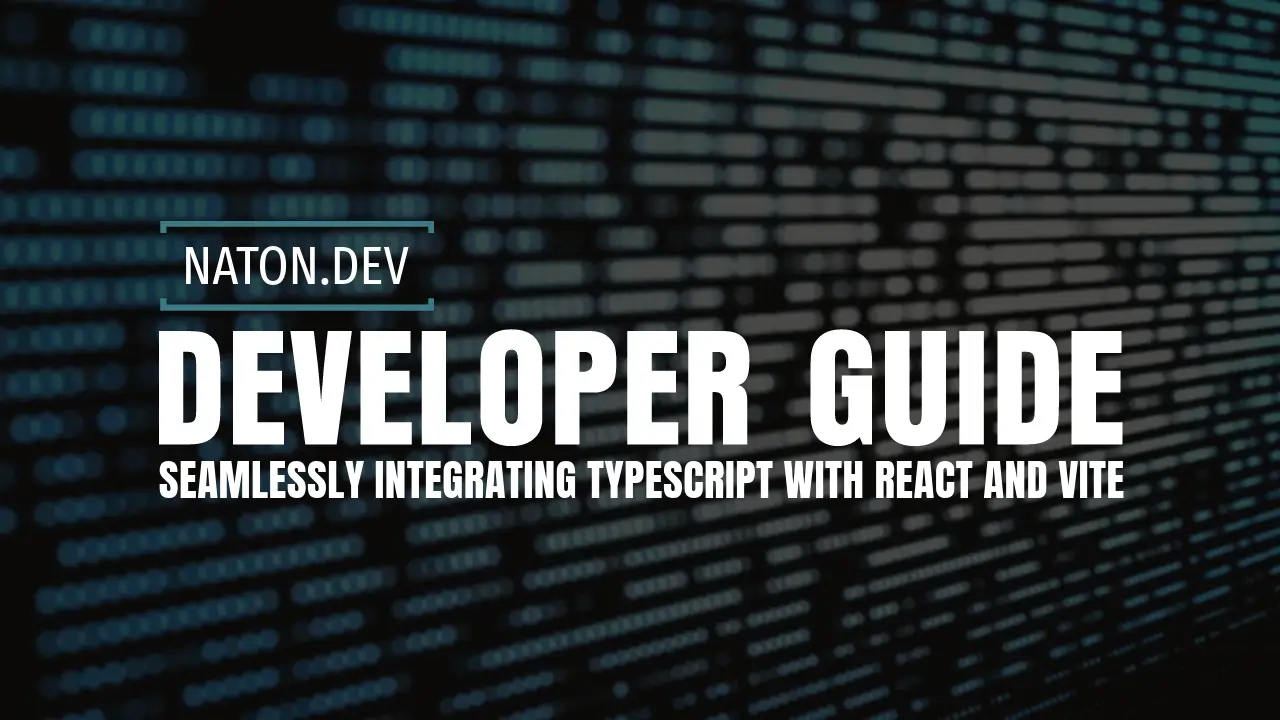
In the evolving landscape of web development, TypeScript has emerged as a powerful tool for building more reliable and maintainable applications. By integrating TypeScript with React, developers can enjoy an enhanced development experience with static type checking, reducing runtime errors and improving code quality. When combined with the lightning-fast build tool Vite, the development workflow becomes even more efficient and enjoyable. This guide will walk you through the process of integrating TypeScript with React in a Vite project, step by step.
Why TypeScript with React?
TypeScript, a superset of JavaScript, brings static typing to the dynamic world of JavaScript. This means errors can be caught at compile time rather than at runtime, leading to more robust code. When used with React, TypeScript improves the development experience by enabling intelligent code completion, early error detection, and readable code, especially in large-scale applications.
Getting Started
Before diving into the integration, ensure you have Node.js installed on your system. This guide assumes you have basic knowledge of React and TypeScript.
Step 1: Create a New Vite Project with TypeScript
To get started, open your terminal and run the following command to create a new React project with Vite and TypeScript:
npm create vite@latest my-react-ts-app -- --template react-ts
This command creates a new directory named my-react-ts-app
with a React + TypeScript template. Navigate into your new project directory:
cd my-react-ts-app
Step 2: Explore the Project Structure
The project setup by Vite includes several configuration files and a basic project structure:
index.html
: The entry point for your application.src/main.tsx
: The main TypeScript file where your React app is initialized.src/App.tsx
: A simple React component written in TypeScript.tsconfig.json
: The TypeScript configuration file.
Step 3: Understand tsconfig.json
The tsconfig.json
file in your project root defines options required to compile your project. Vite's template comes with a pre-configured tsconfig.json
suited for React and TypeScript projects. You might want to review and adjust these settings as your project grows.
Step 4: Writing TypeScript with React
Open src/App.tsx
to see a React component written in TypeScript. Notice how TypeScript allows you to define props and state with specific types. For instance:
interface AppProps {
title: string;
}
function App({ title }: AppProps) {
return <div>{title}</div>;
}
This snippet demonstrates a functional component App
with typed props using an interface.
Step 5: Running Your Project
To run your project, use the following command:
npm run dev
Your application should now be running on http://localhost:3000
. You'll see the benefits of TypeScript as you develop, with your editor providing autocompletion and type checking in real-time.
Advantages of Using TypeScript with React and Vite
- Improved Developer Experience: TypeScript's static typing adds a layer of documentation to your code, making it easier to understand and maintain.
- Enhanced Code Quality: Early error detection and type checking reduce runtime errors.
- Fast Development Cycle: Vite offers out-of-the-box TypeScript support, ensuring fast refresh and build times.
Best Practices
- Define Component Props and State Clearly: Use interfaces or types for props and state to leverage TypeScript's full potential.
- Gradually Adopt TypeScript: If integrating TypeScript into an existing React project, start by converting components incrementally.
- Leverage TypeScript Features: Utilize enums, generics, and other TypeScript features to write concise and type-safe code.
Conclusion
Integrating TypeScript with React and Vite offers a modern, efficient, and safe development environment. This guide provided the steps to start a new project with these technologies, emphasizing the importance of type safety and the advantages it brings to your development workflow. As you continue to build with React, TypeScript, and Vite, you'll find that this combination not only speeds up the development process but also enhances the quality of your web applications.