The Power of Serverless Computing with AWS Lambda and Node.js
Unleash the full potential of serverless computing: Dive into AWS Lambda with Node.js and transform how you build, deploy, and scale your applications effortlessly.
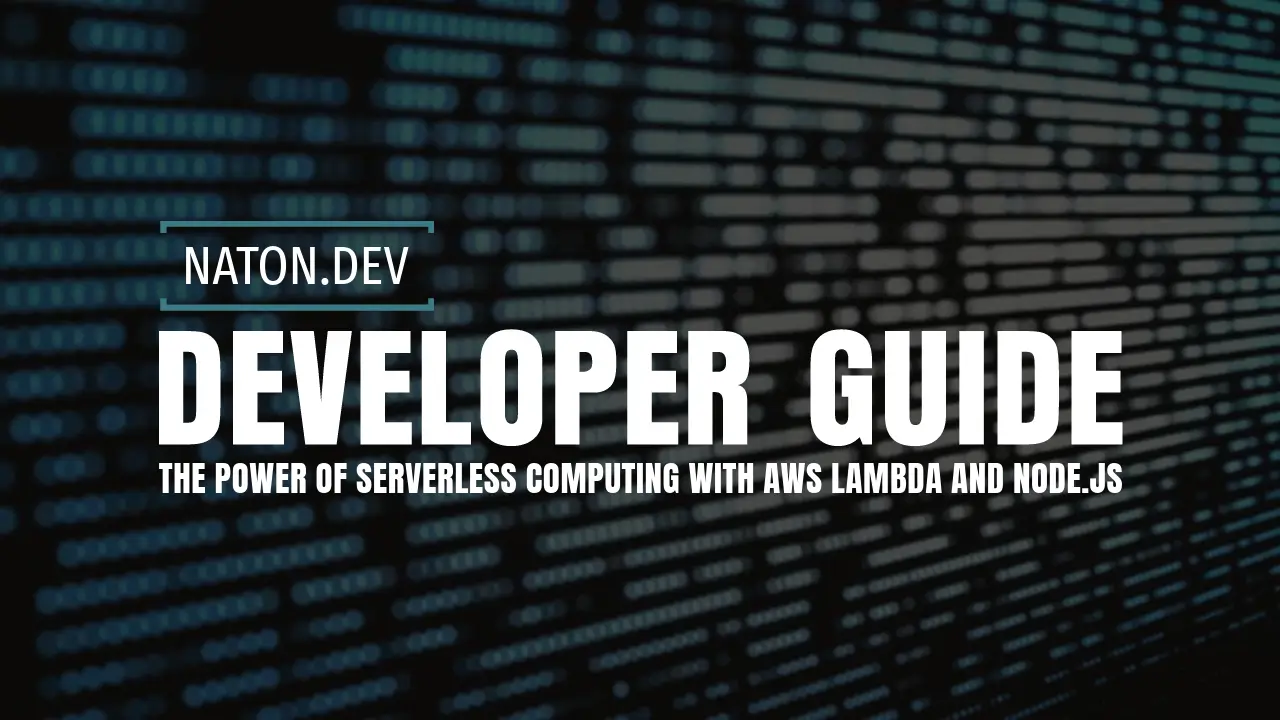
The rise of serverless computing has marked a significant shift in how developers build and deploy applications. This paradigm allows for greater scalability, cost efficiency, and development agility. AWS Lambda, a cornerstone of Amazon Web Services' serverless offerings, enables developers to run code in response to events without provisioning or managing servers. When combined with Node.js, AWS Lambda becomes an even more powerful tool for creating efficient, scalable applications. This article explores how to leverage AWS Lambda with Node.js, guiding you from the basics to more advanced practices.
Introduction to AWS Lambda and Node.js
Overview of Serverless Computing
Serverless computing is an execution model where the cloud provider manages the execution environment, dynamically handling the provisioning of resources. This model allows developers to focus solely on writing code, significantly reducing the overhead of server management, scaling, and maintenance.
What is AWS Lambda?
AWS Lambda is a service that runs your code in response to triggers such as HTTP requests, database events, or queue operations. It automatically manages the compute resources, scaling your application with the size of the workload.
Benefits of Using AWS Lambda with Node.js
Combining AWS Lambda with Node.js offers several advantages:
- Scalability: Automatically scales with the application's needs.
- Cost-Effectiveness: Charges are based only on the compute time used.
- Rapid Development: The Node.js environment is conducive to quick development cycles.
- Versatility: Ideal for I/O-bound tasks and asynchronous operations.
Getting Started with AWS Lambda and Node.js
Setting Up an AWS Account
Begin by creating an AWS account at the AWS homepage. Follow the registration process, which includes payment information and identity verification.
Introduction to the AWS Management Console
The AWS Management Console is your portal to managing AWS services. It provides an intuitive interface for deploying, monitoring, and managing resources.
Creating Your First Lambda Function with Node.js
- Step-by-Step Guide:
- Navigate to the Lambda section in the AWS Management Console.
- Click "Create function" and select "Author from scratch."
- Name your function and choose Node.js as the runtime.
- Understanding the AWS Lambda Interface:
The interface allows you to configure triggers, edit code, and set environment variables directly in the browser.
Writing Your First Node.js Function:
exports.handler = async (event) => {
return 'Hello, World!';
};
Deploy and test your function using the console's test feature.
Deep Dive into AWS Lambda Features
Event Sources
AWS Lambda can be triggered by various sources such as S3 buckets, DynamoDB tables, or API Gateway requests. This flexibility allows Lambda to serve as a powerful connector within your architecture.
Environment Variables
Manage configuration and secrets efficiently using environment variables, keeping your Lambda functions secure and modular.
IAM Roles and Permissions
Secure your Lambda functions by defining IAM roles that specify the permissions each function has within your AWS environment.
Monitoring and Logging with CloudWatch
AWS CloudWatch provides monitoring and logging capabilities, essential for tracking the performance and health of your Lambda functions.
Best Practices for AWS Lambda with Node.js
Writing Efficient Lambda Functions
Focus on code optimization, appropriate memory allocation, and configuring timeouts to ensure your Lambda functions are efficient and cost-effective.
Deployment Strategies
Utilize the AWS CLI for manual deployments or automate your deployment process using tools like AWS SAM or the Serverless Framework.
Error Handling and Debugging
Implement robust error handling and leverage CloudWatch logs to debug and optimize your Lambda functions.
Advanced Topics in AWS Lambda with Node.js
Working with External Libraries and Dependencies
AWS Lambda with Node.js allows developers to take advantage of the npm ecosystem, which hosts a vast array of libraries and modules. By incorporating these external libraries into your Lambda functions, you can significantly enhance their functionality, streamline the development process, and avoid "reinventing the wheel" for common tasks. To use an external library, you simply include it in your project's package.json
file and deploy it along with your Lambda function. AWS Lambda's runtime environment automatically resolves these dependencies when your function is invoked, ensuring that your code has access to all the necessary libraries. However, it's essential to be mindful of the package size and deployment limits, optimizing dependencies to avoid bloating your Lambda function and potentially increasing cold start times.
Lambda Layers
Lambda Layers are a powerful feature that allows developers to manage and deploy shared code and libraries across multiple Lambda functions. Instead of packaging and deploying the same library with every function, you can create a Lambda Layer containing the shared code or libraries and reference it in your functions. This approach not only reduces the size of your function deployments but also simplifies updates and management of shared code. If a library needs to be updated or modified, you can do so in the Layer, and all functions referencing that Layer will automatically have access to the updated code. Lambda Layers support versioning, enabling you to easily manage changes and roll back if necessary.
VPC Integration
AWS Lambda's integration with Amazon Virtual Private Cloud (VPC) allows your Lambda functions to access resources within a private network, such as databases, cache instances, or internal services. This capability is crucial for applications that require secure access to private resources or need to interact with other services within a VPC. When you configure a Lambda function to connect to a VPC, AWS sets up elastic network interfaces within your VPC, enabling your function to communicate with other resources securely. While VPC integration opens up new possibilities for serverless applications, it's important to understand the potential impact on cold start times and to implement best practices for network configuration and security.
Cold Start Optimization
Cold starts refer to the latency experienced when invoking a Lambda function after it has been idle for some time, requiring AWS to initialize a new instance of the function. Cold start times can vary based on the runtime, memory configuration, and whether the function needs to establish a connection to a VPC. To minimize the impact of cold starts, developers can implement several strategies, such as keeping functions warm by invoking them periodically, optimizing function code and dependencies to reduce initialization time, and utilizing Provisioned Concurrency, a feature that allows you to keep a specified number of instances warm and ready to serve requests. Another strategy involves optimizing the deployment package size and using Lambda Layers for shared libraries to reduce the amount of data that needs to be loaded during initialization.
By understanding and leveraging these advanced topics, developers can build more efficient, scalable, and powerful serverless applications with AWS Lambda and Node.js, taking full advantage of the cloud's flexibility and capabilities.
Real-world Use Cases
Building RESTful APIs
AWS Lambda, when combined with Amazon API Gateway, provides a powerful, scalable way to build serverless RESTful APIs. This integration allows developers to create APIs that can easily scale to handle a vast number of requests without the need to manage any servers. The API Gateway acts as the front door for requests to access your Lambda functions. This setup not only simplifies the deployment and management of APIs but also enables developers to implement a variety of authorization and authentication mechanisms, including OAuth, JWT tokens, and AWS IAM roles. This approach is particularly beneficial for mobile backends, web application backends, and microservices.
Processing Data Streams
Lambda's ability to process and analyze streaming data in real-time is another compelling use case, especially when integrated with Amazon Kinesis. This setup enables developers to write functions that react to data streams, such as logs, event data, or social media feeds, allowing for the implementation of real-time analytics and dashboarding, data cleansing, metrics generation, and more. For instance, a Lambda function can be triggered for each new record added to a Kinesis stream, processing the data on the fly and storing the results in databases, making it immediately available for business intelligence tools.
Automating Workflows
AWS Step Functions and AWS Lambda can work together to automate complex workflows. Step Functions allow you to design and execute workflows that stitch together services like AWS Lambda, ensuring that each step in your application executes in order, as expected, and is retried when errors occur. This setup is ideal for data processing pipelines, IT automation tasks, and complex ETL jobs. For example, a workflow could automatically trigger Lambda functions to process uploaded images, perform image recognition, store metadata in a database, and then notify users—all without requiring manual intervention or complex code to manage the workflow logic.
Each of these use cases demonstrates the flexibility and power of AWS Lambda within the AWS ecosystem, highlighting how serverless computing can be leveraged to build efficient, scalable, and cost-effective solutions across various domains.
Conclusion
AWS Lambda and Node.js are a potent combination for serverless application development, offering scalability, cost-efficiency, and rapid development. By understanding the basics and exploring advanced features, developers can harness the full potential of serverless computing to build innovative and efficient applications.
Additional Learning Resources
- AWS Lambda Official Documentation: Dive into the official resource for all things AWS Lambda, providing comprehensive guides, API references, and best practices. Visit AWS Lambda Documentation
- Node.js AWS SDK: Explore the official SDK to seamlessly integrate your Node.js applications with AWS services. Check out the Node.js AWS SDK
- In-depth Tutorials and Guides: Find a wealth of knowledge through tutorials and courses tailored for AWS Lambda and serverless development across platforms like Udemy, Coursera, and freeCodeCamp. Start learning on freeCodeCamp
- Community Forums and Discussions: Connect with fellow developers on platforms such as the AWS Developer Forums and Stack Overflow to exchange ideas, solve problems, and share experiences. Join the discussion on AWS Developer Forums
- Workshops and Webinars: Enhance your skills by attending AWS-organized events and webinars that offer practical insights into serverless technologies. Explore AWS Events and Webinars
These resources are designed to support your journey from understanding the basics of AWS Lambda and Node.js to mastering advanced serverless architectures, ensuring you have the tools and knowledge to excel in your development projects.