Exploring the React Ecosystem: Hooks, Context, and Router
Uncover the power of Hooks, Context, and Router to build seamless, scalable web applications with ease!
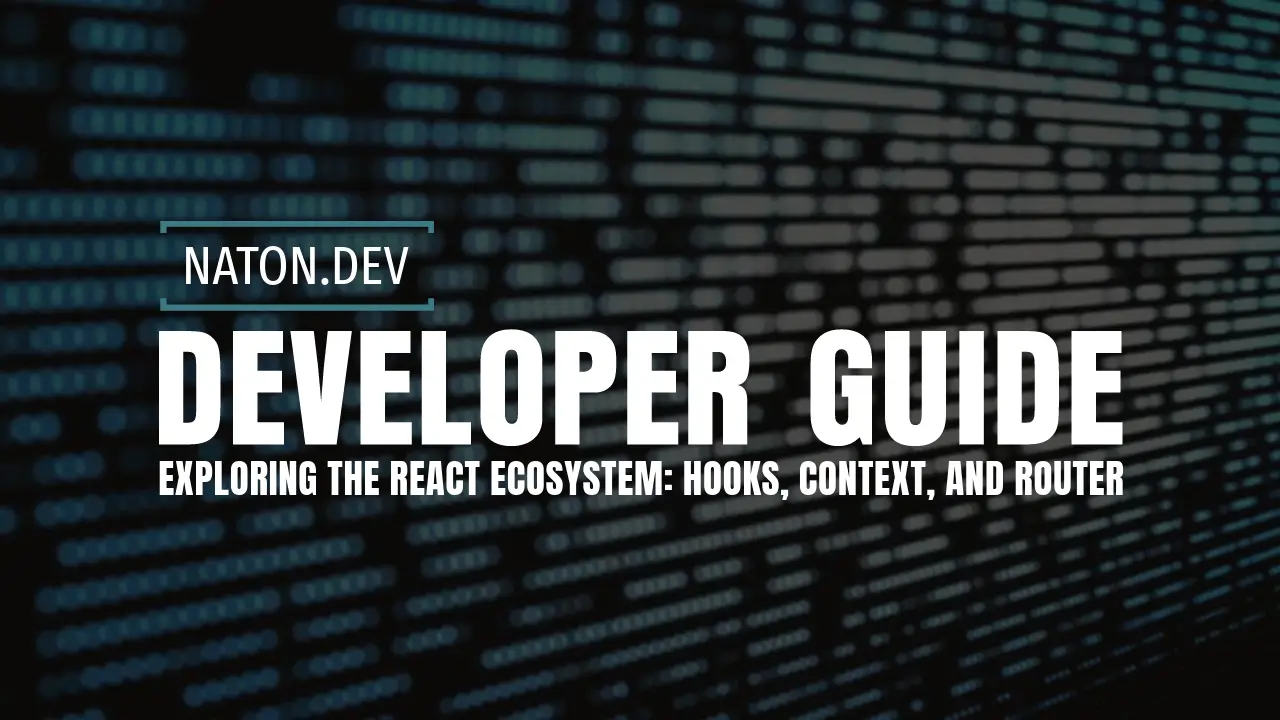
In the vibrant world of web development, React continues to stand out as a library of choice for developers looking to build dynamic and responsive user interfaces. Beyond the basics of creating components and managing state, React offers a rich ecosystem that includes Hooks, Context, and Router. These tools provide powerful solutions for common challenges in web development, such as state management across components, efficient data passing, and client-side routing. This blog post delves into these three pivotal features, offering insights on how to leverage them to enhance your React applications.
React Hooks: State and Lifecycle Management Reimagined
React Hooks, introduced in React 16.8, allow you to use state and other React features without writing a class. Hooks simplify the logic and code organization, making your components cleaner and easier to understand. Here are a few commonly used Hooks:
- useState: This Hook lets you add React state to function components. It returns a stateful value and a function to update it, thereby encapsulating local state management in functional components.
- useEffect: This Hook lets you perform side effects in function components, such as fetching data, subscriptions, or manually changing the DOM. It serves the same purpose as
componentDidMount
,componentDidUpdate
, andcomponentWillUnmount
in React class lifecycle methods. - useContext: This Hook allows you to access the value of a React Context from within a function component. It simplifies the process of passing data through the component tree without having to pass props down manually at every level.
Example: Using useState and useEffect
import React, { useState, useEffect } from 'react';
function Example() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
});
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
React Context: Prop Drilling No More
React Context provides a way to pass data through the component tree without having to pass props down manually at every level. It's particularly useful for global data that needs to be accessible by many components in an application, such as theme settings or user authentication status.
Example: Using React Context
import React, { useContext, useState } from 'react';
const ThemeContext = React.createContext();
function App() {
const [theme, setTheme] = useState('light');
return (
<ThemeContext.Provider value={{ theme, setTheme }}>
<Toolbar />
</ThemeContext.Provider>
);
}
function Toolbar(props) {
return (
<div>
<ThemedButton />
</div>
);
}
function ThemedButton() {
const { theme, setTheme } = useContext(ThemeContext);
return <button onClick={() => setTheme(theme === 'light' ? 'dark' : 'light')}>Change Theme</button>;
}
React Router: Navigating Single Page Applications
React Router is a standard library for routing in React. It enables the navigation among views of various components in a React Application, allows changing the browser URL, and keeps UI in sync with the URL.
Example: Basic Routing with React Router
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import Home from './Home';
import About from './About';
function App() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</Switch>
</Router>
);
}
Conclusion
The React ecosystem offers a wealth of tools and features that can significantly streamline the process of developing complex and interactive web applications. By understanding and integrating Hooks, Context, and Router into your projects, you can build more efficient, scalable, and maintainable applications. Whether you're managing local state with Hooks, avoiding prop drilling with Context, or implementing seamless navigation with React Router, these features are invaluable for any React developer looking to elevate their web development skills.